Alphabet: 26 Languages
A few months ago, I started a project called Alphabet. 26 letters in my alphabet, 26 languages to discover.
What
I intend to write a small (tiny) program in 26 different languages, one for each letter of the latin alphabet.
I’m not sure yet what the program has to do but each implementation will probably have to do something with the first letter of its language.
Why
- I will discover crazy languages
- I will learn a lot about programming languages in general
- It’s fun!
Letters already done
So far, only letters B and L are completed : Brainfuck and Lua.
Brainfuck
If you’ve read my brainfuck series, you’ve already seen the B entry.
1 | +++++++++++[->+++>++++++>+<<<]>->>->>>++>+>+>++>+>+>++<<<<<< |
It prints a big B letter made of little B characters:
BBBB B B B B BBBB B B B B BBBB
Here is the commented code:
1 | *************** |
Lua
The Lua code is a lot easier to read!
I used a framework but it starts with a L (Löve2D) so I decided it was fine :-). It’s a 2D game framework which I intend to write about later.
This code just draws a spinning colored L. Rotation speed and color oscillate.
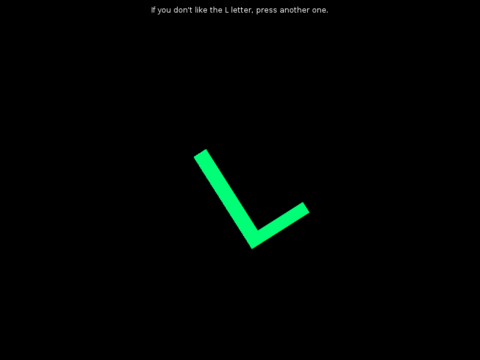
You can change the letter by pressing another one.
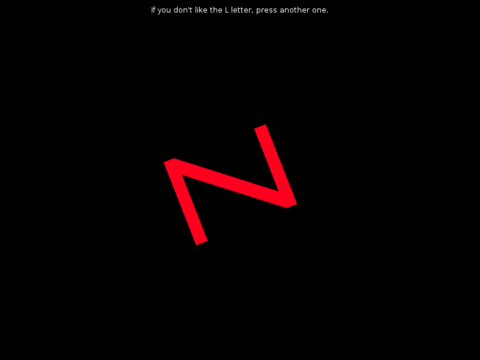
Löve2D calls love.load()
once, then calls love.update()
and love.draw()
repeatedly until you exit the program. love.keypressed/keyreleased()
are
called when such an event occurs.
1 | -- Lua starts with a L |
Next
My goal is to write one program per letter. It’s likely to take years.
I have selected several candidates for the other letters. Some are well-known languages, some are esoteric.
- A: APL, Ada
- C: Clojure,
- E: Erlang
- F: FALSE
- G: Go, Groovy
- H: Haskell
- I: Io
- P: Piet, PostScript, Prolog
- R: Ruby
- S: Scala, Scheme, Self
- T: Thue
- V: Vala
- X: XSLT
2 down, 24 to go!